ES7 新特新
Array.prototype.includes
includes 方法用于检测数组中是是否包含某一个元素,返回布尔类型值。类似于typeof
但是返回值是数字,因此引入includes ,对判断更加方便
指数操作符
在 ES7 中引入指数运算符 【**】,用来实现幂运算,功能与 Math.pow
相同
console.log(2 ** 10) //logs 1024 ,2的10次方
console.log(Math.pow(2,10))
ES8 新特新
ES8 引入了 async
await
两种语法,结合可以让异步代码像同步代码一样
async 函数
- async 函数返回值为 promise 对象
- promise 对象的结果由 async 函数执行的返回值决定
1 2 3 4 5 6 7 8 9 10 11 12
| async function fn(){ return 'SimpleLife' throw new Error('错误') return new Promise((resolve,reject)=>{ resolve('成功数据') }) }
|
await 表达式
- await 必须写在 async 函数中
- await 右侧的表达式一般为 promise 对象
- await 返回的是 promise 成功的值
- await 的 promise 失败了,就会抛出异常,需要通过 try … catch 捕获异常
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| const p = new Promise((resolve,reject)=>{ reject('失败了') })
async function fn(){ try{ let result = await p; console.log(result) } catch(e){ console.log(e) } }
fn();
|
对象方法的扩展
Object.values
1 2 3 4 5 6 7 8 9
| const simplelife(){ name: 'simplelife', age: 18, height: '1.88' }
let keysname = Object.keys(simplelife)
let values = Object.values(simplelife)
|
Object.entries
返回结果是一个数组,每一个成员也是数组。数组第一个是键名,第二个是值
Object.getOwnPropertyDescriptors
返回结果是对象属性的描述对象
1 2 3 4 5 6 7 8 9 10 11
| const obj = Object.create(null,{ name:{ value: 'SimpleLife', writable: true, configurable: true, enumberable: true } })
|
ES9 新特新
rest 参数与 spread 扩展运算符在 ES6 中已经引入,不过 ES6 中只针对于数组,在 ES9 中为对象提供了像数组一样的 rest 参数和 spread 扩展运算符
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| function connect({host,port,username,password}){ console.log(host) console.log(port) console.log(username) console.log(password) } connect({ host: '127.0.0.1', port: 3306, username: 'root', password: 'root' })
function connect({host,port,...user}){ console.log(host) console.log(port) console.log(user) }
|
1 2 3 4 5 6 7 8 9 10 11 12
| const one = { one: 'oneoneone' } const two = { two: 'twotwotwo' }
const three = { ...one,...two }
|
正则扩展:命名捕获分组
1 2 3 4 5 6 7 8 9 10 11 12
| let blogurl = '<a href="http://www.noti.top">SimpleLife</a>'
const reg = /<a href="(.*)">(.*)<\/a>/
const result = reg.exec(blogurl)
console.log(result[1]) console.log(result[2])
|

1 2 3 4
| const reg = /<a href="(?<url>.*)">(?<text>.*)<\/a>/
|
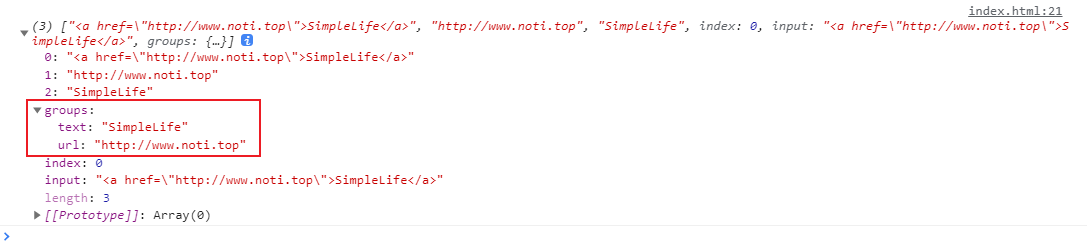
正则扩展:反向断言
1 2 3 4 5 6 7 8
| let str = 'JS5211314你知道么555啦啦啦' const reg = /\d+(?=啦啦啦)/ const result = reg.exec(str) console.log(result)
const reg = /(?<=么)\d+/
|
正则扩展:dotAll 模式
通过 .*
能够匹配任意字符,.*?
只匹配一次
1 2 3 4 5 6 7 8 9 10
| const reg = /<li>.*?<a>(.*?)<\/a>.*?<p>(.*?)<\/p>/s;
const reg = /<li>.*?<a>(.*?)<\/a>.*?<p>(.*?)<\/p>/sg;
while(result = reg.exec(str)){ }
|
正则扩展:.* 和 .*? 的区别
表达式 .* 就是单个字符匹配任意次,即贪婪匹配。
表达式 .*? 是满足条件的情况只匹配一次,即最小匹配.
ES10 新特新
Object.fromEntries
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| const result = Object.fromEntries([ ['name','simplelife'], ['blog','JS,HTML,CSS'] ])
console.log(result);
const m = new Map() m.set('name','Simplelife') const result2 = Object.fromEntries(m) console.log(result2)
const arr = Object.entries({ name: 'Simple' }) console.log(arr)
|
trimStart 与 trimEnd:指定清除左侧和右侧的空白
1 2 3
| let str = ' simplelife ' console.log(str.trimStart()) console.log(str.trimEnd())
|
数组扩展
flat
1 2 3 4 5 6 7 8 9
| const arr = [1,2,3,[5,6]] console.log(arr.flat())
const arr = [1,2,3,[5,6,[7,8,9]]] console.log(arr.flat())
const arr = [1,2,3,[5,6,[7,8,9]]] console.log(arr.flat(2))
|
flatMap
1 2 3 4
| const arr = [1,2,3,4] const result = arr.map(item => [item*10])
const result = arr.flatMap(item => [item*10])
|
Symbol 扩展
Symbol.prototype.description
1 2 3
| let s = Symbol('SimpleLife') console.log(s.description)
|
ES11 新特性
私有属性
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| class Person{ name; #age; #weight; constructor(name,age,weight){ this.name = name this.#age = age this.#weight = weight } }
const girl = new Person('xiaohong',18,'45kg')
console.log(girl.#age) console.log(girl.#weight)
|
Promise.allSettled
1 2 3 4 5 6 7 8 9 10
| const p1 = new Promise(()={})
const p2 = new Promise(()={})
const result = Promise.allSettled([p1,p2])
const res = Promise.all([p1,p2])
|
可选链操作符:?.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| function main(config){ const dbHost = config?.db?.host console.log(dbHost) } main({ db:{ host: '192.168.1.100', username: 'root' }, cache:{ host: '192.168.1.200', username: 'admin' } })
|
动态加载 import
1 2 3 4 5 6 7 8
| const btn = document.getElementById('btn')
btn.onclick = function(){ import('./hello.js').then(module =>{ module.hello() }) }
|
新数据类型 BigInt
1 2 3 4 5 6 7
| let n = 521n console.log(n,typeof(n))
let n = 123 console.log(BigInt(n))
|
应用
1 2 3 4 5 6 7 8 9 10 11
| let max = Number.MAX_SAFE_INTEGER console.log(max) console.log(max+1) console.log(max+2)
console.log(BigInt(max)) console.log(BigInt(max) + BigIng(1)) console.log(BigInt(max) + BigIng(2))
|
globalThis 始终指向全局 this